|
Differences between cfQueryParam "cf_sql_date" and "cf_sql_timestamp" |
I was recently looking at a report generator, and came across a conflict in the mySql query dealing with the date objects.
The report returns a set of records between two dates, using cfqueryparam, but there seemed to be a problem in running the query using certain dates.
Running it from a browser provided differing results from running it directly in a sql interface. So where was the error?
The original code was using the "cf_sql_date" type to pass the date time off to the sql engine. It appears that this data type is not transferred as a date time value, but as an incompatible numeric value, that is not comparable to the mySql datetime I am querying against.
2
3AND table.ap_enddate <= <cfqueryparam cfsqltype="CF_SQL_DATE" value="#createodbcdate(arguments.rangeto)#">
There are a wealth of articles online about passing date values into a variety of database technologies, but this Adobe post was very helpful.
This article shows a handy table of cfsqltypes and how they map to different database management systems.
Using something like sql profiler you can clearly see the difference between cf_sql_date and cf_sql_timestamp, where cf_sql_date is being truncated to just a date value (actually evaluates as a numeric value) and a full date time stamp that cf_sql_timestamp generates.
2
3AND table.ap_enddate <= <cfqueryparam cfsqltype="cf_sql_timestamp" value="#createodbcdate(arguments.rangeto)#">
Changing the code to the above example completely resolves this issue.
|
Ever needed to make a template 'sleep' for a defined period? |
One of the code snippets I've had scattered around is a short java command to make the current thread sleep, for a set period.
Its handy if you want to add a set period of delay to an application, for any reason.
2
3thread = createObject("java", "java.lang.Thread");
4thread.sleep(javaCast("long", 5000));
5
6</cfscript>
It creates the java thread object, and uses the sleep method to pause the thread for whatever numeric value you give it. In this example 5000ms.
|
Non selectable drop down options in forms |
I was recently developing an application that had several select fields in a form. The first dynamically populated the second, but with differing sets of data, that needed to be within the same select box, but seperated in some way.
It was only then that I found there is a 'optgroup' html tag for select boxes! This tag is used to group together related options in a select list. It automatically bolds the text, and is unselectable.
2<cfset variables.mySecondlist = "5,6,7,8">
3
4<cfoutput>
5<select name="number">
6<cfloop list="#variables.mylist#" index="I">
7 <option value="#I#">#I#</option>
8</cfloop>
9
10<optgroup label="Second List"></optgroup>
11
12<cfloop list="#variables.mySecondlist#" index="I">
13 <option value="#I#">#I#</option>
14</cfloop>
15
16</select>
17
18</cfoutput>
Just insert it where you want your category break to be, and assign it some text.
Handy for breaking up large sections of options.
|
Consuming 360 Voices XML data feeds - 360 Voice part 2 |
2<cfset variables.filepath = GetBaseTemplatePath()>
3<cfset variables.filepath = replace(variables.filepath, 'include.cfm', '', 'all')>
4<cfset variables.todaysfile = variables.filepath & "tmp\#DateFormat(NOW(), 'dd-mm-yyyy')#.xml">
2 <!--- Read local file --->
3 <cffile action="read" file="#variables.todaysfile#" variable="xmlfile">
4 <cfscript>
5 xmlfile = xmlparse(xmlfile);
6 </cfscript>
7<cfelse>
8 <cfhttp url="http://www.360voice.com/api/blog-getentries.asp?tag=ect0z" method="GET" charset="utf-8">
9 <cfhttpparam type="Header" name="Accept-Encoding" value="deflate;q=0">
10 <cfhttpparam type="Header" name="TE" value="deflate;q=0">
11 </cfhttp>
12 <cfscript>
13 xmlfile = xmlparse(cfhttp.filecontent);
14 </cfscript>
15 <cffile action="write" file="#variables.todaysfile#" output="#xmlfile#">
16</cfif>
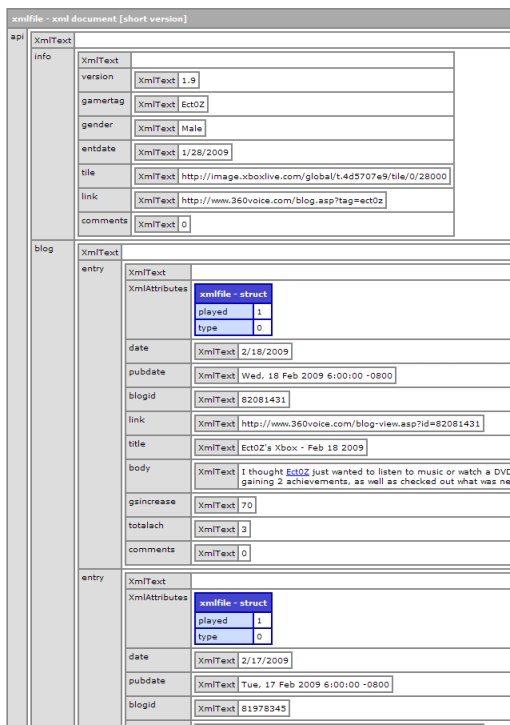
2<cfoutput>
3 <cfloop index="i" from="1" to="#ArrayLen(arrHeader)#">
4 <img src="#arrHeader[i].tile.XmlText#" alt="Gamer Icon"> - 360 Voice.com Blog
5 <!--- #arrHeader[i].link.XmlText# --->
6 </cfloop>
7</cfoutput>
2<cfoutput>
3 <cfloop index="i" from="1" to="#ArrayLen(arrEntries)#">
4 <b>#arrEntries[i].title.XmlText# -#arrEntries[i].date.XmlText#</b><br/>
5 #arrEntries[i].body.XmlText#
6 <p><br/></p>
7 </cfloop>
8</cfoutput>